Enter your email for Morphic updates:
What is Morphic?
Morphic is an experimental pure functional programming language designed to achieve performance competitive with imperative systems languages like C++ and Rust.
Morphic features three automatic optimizations which turn functional programming constructs into zero-cost abstractions:
- Lambdas: Morphic’s lambda set specialization makes lambdas unboxed and statically dispatched, and allows calls to lambdas to be inlined.
- Immutable Data Structures: Morphic’s mutation optimization transforms updates to logically-immutable data structures into in-place mutations when doing so does not affect semantics.
- Automatic Memory Management: Morphic uses a borrow-based reference counting scheme which is able to eliminate almost all reference count increments and decrements for a large class of programs.
type Primality { Prime, Composite, } sieve(limit: Int): Array Primality = // Array memory is automatically managed statically, // without any refcounting overhead in this case. let init_arr = Array.fill(limit, Prime) |> Array.set(0, Composite) |> Array.set(1, Composite) in // Iterator logic compiles to a simple loop, with // no heap allocations or virtual dispatch. Iter.range(2, limit) |> Iter.foldl(init_arr, \(arr, n) -> match Array.get(arr, n) { Prime -> Iter.ints(2) |> Iter.map(\i -> i * n) |> Iter.take_while(\i -> i < limit) |> Iter.foldl( arr, // Array updates logically copy the array, // but are automatically performed in-place // when safe. \(new, i) -> Array.set(new, i, Composite) ), Composite -> arr, } )
Contributors
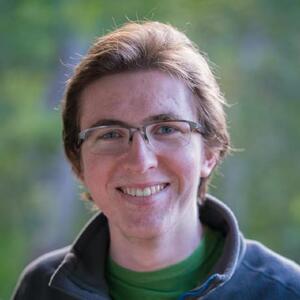
William Brandon
wbrandon@csail.mit.eduPhD student at MIT CSAIL researching programming languages, compilers, and high-performance deep learning.
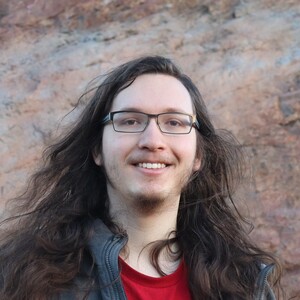
Benjamin Driscoll
bdrisc@cs.stanford.eduPhD student at Stanford researching programming languages and compilers.
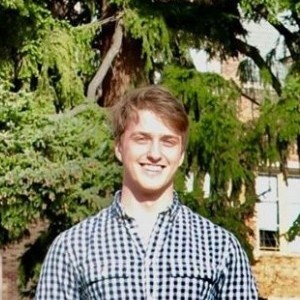
Wilson Berkow
Compiler engineer.
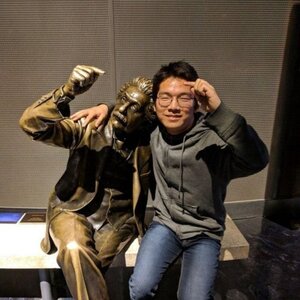
Frank Dai
Type theorist.
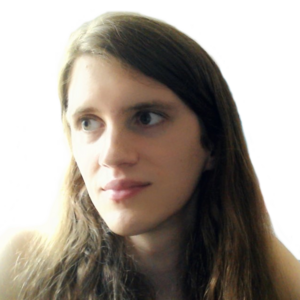
Mae Milano
Assistant professor at Princeton University working in programming language design for systems.